Evolving multiple binaries¶
Following on from evolving a single binary, we can also evolve multiple binaries. Let’s start by importing the necessary modules:
In [1]: from cosmic.sample.initialbinarytable import InitialBinaryTable
In [2]: from cosmic.evolve import Evolve
And use the same BSE dict as before:
In [3]: BSEDict = {'xi': 1.0, 'bhflag': 1, 'neta': 0.5, 'windflag': 3, 'wdflag': 1, 'alpha1': 1.0, 'pts1': 0.001, 'pts3': 0.02, 'pts2': 0.01, 'epsnov': 0.001, 'hewind': 0.5, 'ck': 1000, 'bwind': 0.0, 'lambdaf': 0.0, 'mxns': 3.0, 'beta': -1.0, 'tflag': 1, 'acc2': 1.5, 'grflag' : 1, 'remnantflag': 4, 'ceflag': 0, 'eddfac': 1.0, 'ifflag': 0, 'bconst': 3000, 'sigma': 265.0, 'gamma': -2.0, 'pisn': 45.0, 'natal_kick_array' : [[-100.0,-100.0,-100.0,-100.0,0.0], [-100.0,-100.0,-100.0,-100.0,0.0]], 'bhsigmafrac' : 1.0, 'polar_kick_angle' : 90, 'qcrit_array' : [0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0,0.0], 'cekickflag' : 2, 'cehestarflag' : 0, 'cemergeflag' : 0, 'ecsn' : 2.25, 'ecsn_mlow' : 1.6, 'aic' : 1, 'ussn' : 0, 'sigmadiv' :-20.0, 'qcflag' : 1, 'eddlimflag' : 0, 'fprimc_array' : [2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0,2.0/21.0], 'bhspinflag' : 0, 'bhspinmag' : 0.0, 'rejuv_fac' : 1.0, 'rejuvflag' : 0, 'htpmb' : 1, 'ST_cr' : 1, 'ST_tide' : 1, 'bdecayfac' : 1, 'rembar_massloss' : 0.5, 'kickflag' : 1, 'zsun' : 0.014, 'bhms_coll_flag' : 0, 'don_lim' : -1, 'acc_lim' : -1, 'rtmsflag' : 0, 'wd_mass_lim': 1}
Below is an example for systems that could form GW150914 and GW170817 - like binaries.
In [4]: binary_set = InitialBinaryTable.InitialBinaries(m1=[85.543645, 11.171469], m2=[84.99784, 6.67305],
...: porb=[446.795757, 170.758343], ecc=[0.448872, 0.370],
...: tphysf=[13700.0, 13700.0],
...: kstar1=[1, 1], kstar2=[1, 1],
...: metallicity=[0.002, 0.02])
...:
In [5]: print(binary_set)
kstar_1 kstar_2 mass_1 mass_2 ... bhspin_1 bhspin_2 tphys binfrac
0 1.0 1.0 85.543645 84.99784 ... 0.0 0.0 0.0 1.0
1 1.0 1.0 11.171469 6.67305 ... 0.0 0.0 0.0 1.0
[2 rows x 38 columns]
In [6]: import numpy as np
In [7]: np.random.seed(5)
In [8]: bpp, bcm, initC, kick_info = Evolve.evolve(initialbinarytable=binary_set, BSEDict=BSEDict)
As before, bpp, bcm, and initC are returned as pandas DataFrames which assign an index to each binary system we evolve. We can access each binary as follows:
In [9]: print(bpp.loc[0])
tphys mass_1 mass_2 ... bhspin_1 bhspin_2 bin_num
0 0.000000 85.543645 84.997840 ... 0.0 0.0 0
0 3.717075 72.720332 72.376321 ... 0.0 0.0 0
0 3.718373 72.589218 72.377845 ... 0.0 0.0 0
0 3.720012 71.959504 72.806393 ... 0.0 0.0 0
0 3.740038 32.352601 110.573279 ... 0.0 0.0 0
0 3.741215 32.176152 110.618802 ... 0.0 0.0 0
0 4.071374 25.488585 106.891756 ... 0.0 0.0 0
0 4.071374 24.988585 106.891756 ... 0.0 0.0 0
0 4.894369 24.988590 88.885767 ... 0.0 0.0 0
0 4.895887 24.989628 88.681486 ... 0.0 0.0 0
0 4.896841 24.990676 88.469784 ... 0.0 0.0 0
0 4.896841 24.990676 88.469784 ... 0.0 0.0 0
0 4.896841 24.990676 41.981622 ... 0.0 0.0 0
0 4.896841 24.990676 41.981622 ... 0.0 0.0 0
0 4.906644 25.001441 41.981622 ... 0.0 0.0 0
[15 rows x 44 columns]
In [10]: print(bcm.loc[0])
tphys 0.0
kstar_1 1
mass0_1 85.543645
mass_1 85.543645
lum_1 941613.739772
rad_1 11.060285
teff_1 54306.854457
massc_1 0.0
radc_1 0.0
menv_1 0.0
renv_1 0.0
epoch_1 0.0
omega_spin_1 900.454816
deltam_1 0.0
RRLO_1 0.038788
kstar_2 1
mass0_2 84.99784
mass_2 84.99784
lum_2 930960.003255
rad_2 11.023387
teff_2 54243.142505
massc_2 0.0
radc_2 0.0
menv_2 0.0
renv_2 0.0
epoch_2 0.0
omega_spin_2 903.673613
deltam_2 0.0
RRLO_2 0.038772
porb 446.795757
sep 1363.435508
ecc 0.448872
B_1 0.0
B_2 0.0
SN_1 0
SN_2 0
bin_state 0
merger_type -001
bin_num 0
Name: 0, dtype: object
In [11]: print(initC.loc[0])
kstar_1 1.000000
kstar_2 1.000000
mass_1 85.543645
mass_2 84.997840
porb 446.795757
...
fprimc_11 0.095238
fprimc_12 0.095238
fprimc_13 0.095238
fprimc_14 0.095238
fprimc_15 0.095238
Name: 0, Length: 138, dtype: float64
In [12]: print(bpp.loc[1])
tphys mass_1 mass_2 ... bhspin_1 bhspin_2 bin_num
1 0.000000 11.171469 6.673050e+00 ... 0.0 0.0 1
1 19.426981 10.768477 6.664659e+00 ... 0.0 0.0 1
1 19.461132 10.766344 6.664703e+00 ... 0.0 0.0 1
1 19.476609 10.544935 6.885037e+00 ... 0.0 0.0 1
1 19.476609 10.544935 6.885037e+00 ... 0.0 0.0 1
1 19.476609 2.415521 6.885037e+00 ... 0.0 0.0 1
1 19.476609 2.415521 6.885037e+00 ... 0.0 0.0 1
1 19.492181 2.415521 2.415435e+204 ... 0.0 0.0 1
1 19.515599 2.415521 2.415435e+204 ... 0.0 0.0 1
[9 rows x 44 columns]
The plotting function can also take in multiple binaries. Let’s plot both the GW150914-like progenitor evolution and the GW170817-like progenitor evolutions. For the GW170817-like progenitor, we expect most of the evolution to take place in the first ~60 Myr.
In [13]: fig = evolve_and_plot(binary_set, t_min=None, t_max=[6.0, 60.0], BSEDict=BSEDict, sys_obs={})
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
Cell In[13], line 1
----> 1 fig = evolve_and_plot(binary_set, t_min=None, t_max=[6.0, 60.0], BSEDict=BSEDict, sys_obs={})
NameError: name 'evolve_and_plot' is not defined
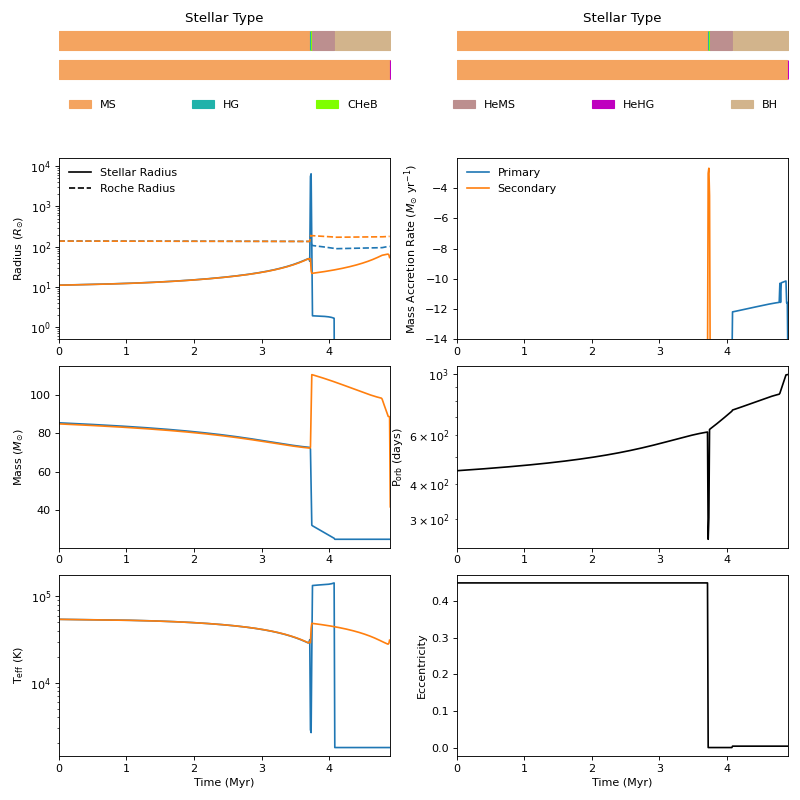
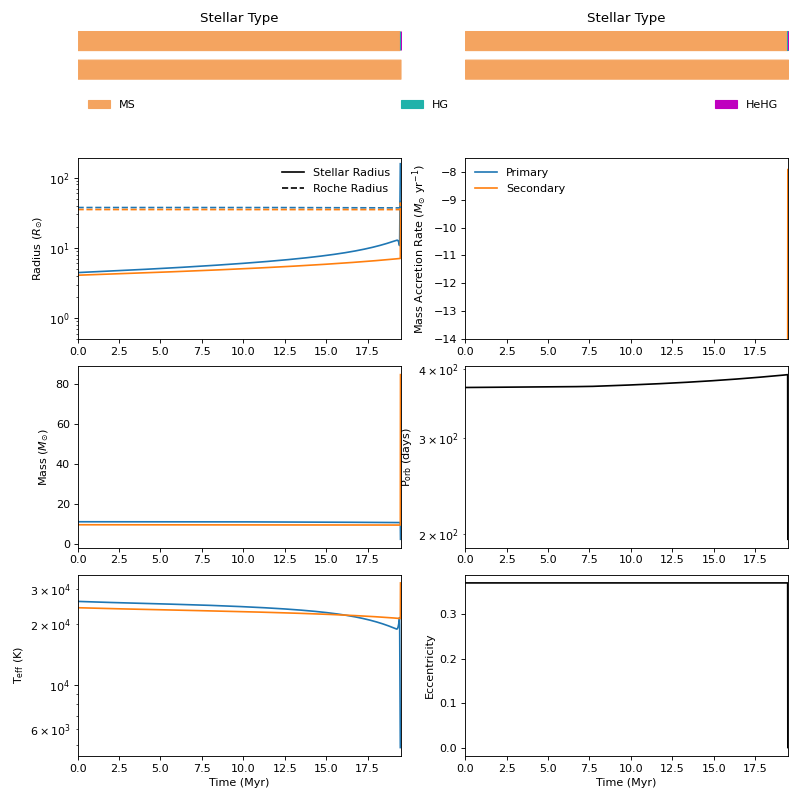